Why is processing a sorted array faster than processing an unsorted array?
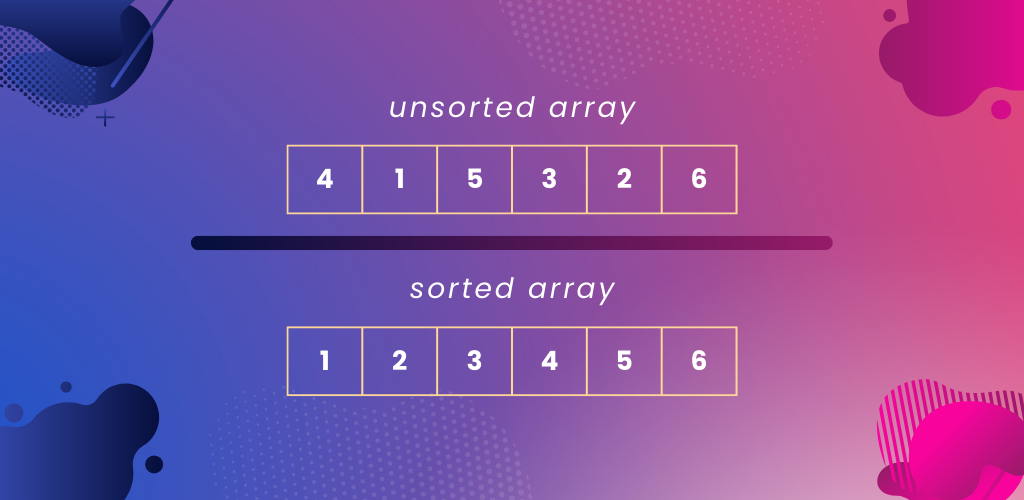
Processing a sorted array can be faster than processing an unsorted array due to improved data locality and the ability to use optimized algorithms. Here’s a brief explanation using Java as requested:
- Data Locality: When an array is sorted, elements are typically stored in a contiguous manner in memory. This enhances data locality, allowing the CPU to fetch data more efficiently from memory caches. In contrast, an unsorted array can lead to frequent cache misses, which slow down processing.
// Sorted array
int[] sortedArray = {1, 2, 3, 4, 5};
// Unsorted array
int[] unsortedArray = {4, 1, 5, 3, 2};
- Optimized Algorithms: Certain algorithms, like binary search, work much faster on sorted data because they can take advantage of the sorted order to eliminate half of the remaining elements in each step. In contrast, searching in an unsorted array typically requires checking each element individually.
// Binary search on a sorted array
int index = Arrays.binarySearch(sortedArray, 3); // Returns index 2
// Linear search on an unsorted array
int index = -1;
for (int i = 0; i < unsortedArray.length; i++) {
if (unsortedArray[i] == 3) {
index = i; // Returns index 3, but takes longer
break;
}
}