What is difference between List Tuple and dictionary In python
In Python, lists, tuples, and dictionaries are three different data structures, each with its own characteristics and use cases. Here’s a brief overview of the differences between them
List
Lists are ordered collections of items.
They are mutable, which means you can change their contents (add, remove, or modify elements) after they are created.
Lists are defined using square brackets ” [ ] “
Example: test_list = [1, 2, 3, 4
]
Tuple
Tuples are ordered collections of items, just like lists.
However, they are immutable, which means once you create a tuple, you cannot change its contents
Tuples are defined using parentheses ()
Example: my_tuple = (1, 2, 3, 4)
Dictionaries
Dictionaries are unordered collections of key-value pairs. Instead of using indices like lists and tuples, you access elements in a dictionary using keys.
Dictionaries are mutable, so you can add, modify, or remove key-value pairs
Dictionaries are defined using curly braces {}
or with the dict()
constructor.
Example: my_dict = {'name': 'Alice', 'age': 30, 'city': 'New York'}
Here’s a comparison table to summarize the differences
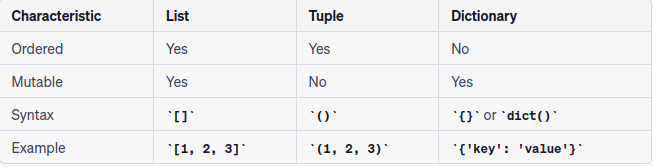
Choose the appropriate data structure based on your specific needs. Use lists when you need an ordered collection of items that can change. Use tuples when you need an ordered collection that should remain constant. Use dictionaries when you need to store key-value pairs and want to quickly access values based on their keys
See What is list on click below
https://onlinecodinghelp.com/index.php/2023/09/06/what-is-list-in-python/