1. There are many Conditional Statement Blogs but Most exciting one is here
A “Conditional Statement” in programming is a fundamental construct that allows a program to make decisions based on certain conditions or criteria. These statements enable a program to execute different sets of instructions depending on whether a specified condition is true or false. What is if in simple word is here.
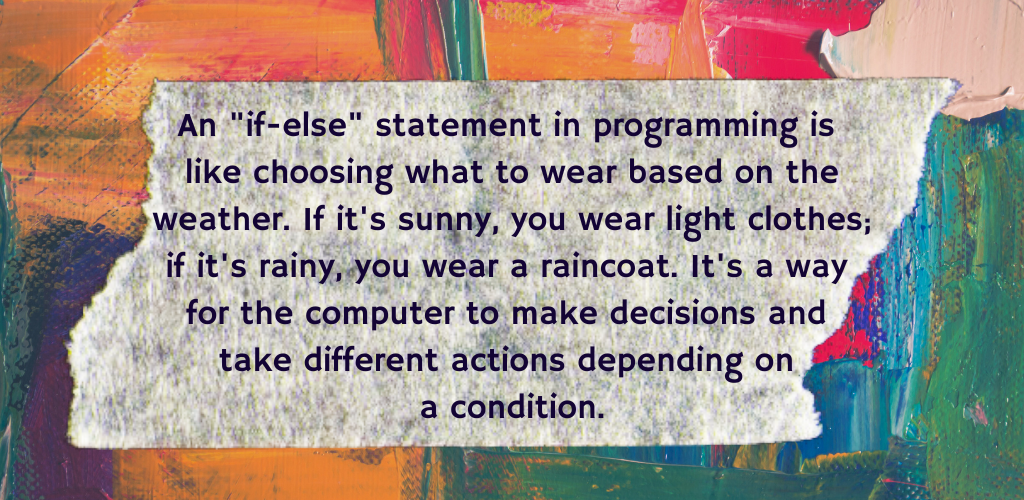
What is if conditional statement?
If statements are generally used as decision making statements. Which is used to execute a block based on the value of the given condition. it is core concept of the any programming language.
Different conditional statements are as below
1. Simple if conditional statement
Syntax of if conditional statement
if(condition/expression){
// Body - Statements inside the block will execute if the given condition is true
}
Flow Chart of If statement :
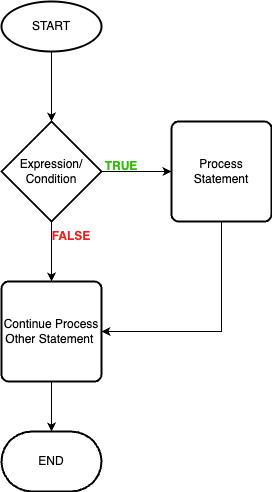
How it works?
Step-1 : Start of the program.
Step-2 : Check the given expression/condition whether it is satisfied or not.
Step-3 : Optional – if the given condition is satisfied then execute block of statement
Step-4 : Continue processing other statements
Step-5 : End of Program
How to use if statement in Java/C/C++ ?
// - Java Programming Example
public class Main {
public static void main(String[] args) {
// TODO: Example of If statement
System.out.println("First Example");
if(5 > 3){ // is 5 more than 3 -- Yes - returns true
System.out.println("5 is bigger than 3");
}
System.out.println("End of first example");
}
}
// - C/C++ programming Example
int main()
{
// TODO: Example of If statement
printf("First Example\n");
if(5 > 3){ // is 5 more than 3 -- Yes - returns true
printf("5 is bigger than 3\n");
}
printf("End of first example");
}
Output :
First Example
5 is bigger than 3
End of First example
When to use?
When a programmer want to execute any block of code based on a condition or conditions then they can use if statement as decision making statement. if the given condition will satisfied then given block of code will be executed and populate result.
Advantages of if statements :
- It’s very easy to use and understand
- It’s simple decision making statement.
- it can evaluate all type of expressions.