1. There are many Conditional Statement Blogs but Most exciting one is here
2. If-Else Statement
if-else statement is worked as either-or condition. if the given condition is true then it will execute block of code else it will execute else block.
Syntax of if-else conditional statement
if(condition/expression){
// Body - Statements inside the block will execute if the given condition is true/correct
}
else{
// Body - Statements inside the block will execute if the given condition is false/wrong
}
Flow chart of If-Else statement
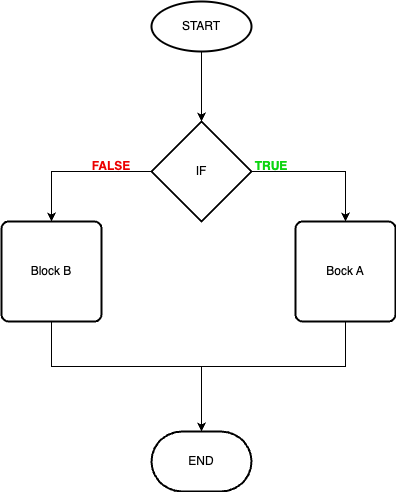
How it works?
Step-1 : Start of the program.
Step-2 : Check the given expression/condition whether it is satisfied or not.
Step-3 : Conditional – if the given condition is satisfied and it return true then execute block A
Step-4 : Conditional – if the given condition is not satisfied and it return false then execute block B
Step-5 : End of Program
How to use if statement in Java/C/C++ ?
// - Java Programming Example
public class Main {
public static void main(String[] args) {
// TODO: Example of If statement
System.out.println("First Example");
if(5 > 3){ // is 5 more than 3 -- Yes - returns true
System.out.println("5 is bigger than 3");
}else{
System.out.println("3 is smaller than 5");
}
System.out.println("End of first example");
System.out.println("---------------------------------");
System.out.println("Second Example");
if(5 < 3){ // is 3 more than 5 -- No - returns False
System.out.println("5 is bigger than 3");
}else{
System.out.println("3 is smaller than 5");
}
System.out.println("End of first example");
}
}
// - C/C++ programming Example
int main()
{
// TODO: Example of If statement
printf("First Example\n");
if(5 > 3){ // is 5 more than 3 -- Yes - returns true
printf("5 is bigger than 3\n");
}else {
printf("3 is smaller than 5\n");
}
printf("---------------------------------\n");
printf("First Example\n");
if(5 > 3){ // is 5 more than 3 -- Yes - returns true
printf("5 is bigger than 3\n");
}else {
printf("3 is smaller than 5\n");
}
printf("End of first example");
}
Output :
First Example
5 is bigger than 3
End of first example
———————————
Second Example
3 is smaller than 5
End of first example
When to use?
When there is a scenario where programmer want to execute block of code based on either-or codition then they can use if-else statement. if the given condition is true then block A will be executed or block B will be executed.