Mastering Loops in Java
Welcome to our comprehensive guide on “Loops in Java.” Whether you’re a beginner or an experienced programmer, this article will take you on a journey through the essential concepts, advantages, and real-world applications of loops in the Java programming language.
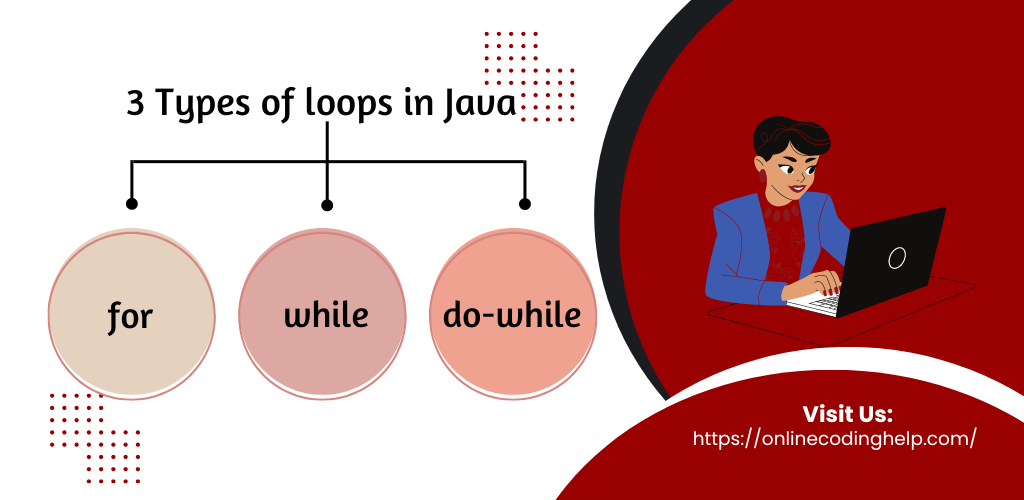
Table of Contents
What is a loop in Java?
Loops are fundamental constructs in programming that allow you to repeat a specific set of instructions multiple times. In Java, loops are indispensable for automating repetitive tasks, iterating through collections of data, and implementing various algorithms efficiently.
What are the 3 types of loops in Java?
Implementing Loops: Step-by-Step
Now that we understand the concept of loops in Java and their advantages, let’s dive into the syntax and usage of the three main types of loops: for
, while
, and do-while
. We’ll also explore loop control statements.
Syntax and Examples
The for Loop
The for
loop is commonly used when you know the number of iterations required. Its syntax consists of three parts within the parentheses:
Syntax:
for (initialization; condition; update) {
// Code to be executed
}
- Initialization: This part is executed only once at the beginning. It’s used to initialize loop control variables.
- Condition: The loop continues executing as long as this condition remains true. If the condition evaluates to false initially, the loop won’t execute.
- Update: This part is executed after each iteration and is typically used to update loop control variables.
Example:
for (int i = 1; i <= 5; i++) {
System.out.println("Iteration " + i);
}
The while Loop
The while
loop continues executing a block of code as long as a given condition remains true. It’s ideal for situations where the number of iterations isn’t predetermined.
Syntax:
while (condition) {
// Code to be executed
}
Example:
int count = 1;
while (count <= 3) {
System.out.println("Iteration " + count);
count++;
}
The do-while Loop
The do-while
loop is similar to the while
loop but guarantees that the code block will execute at least once, even if the condition is false initially.
Syntax:
do {
// Code to be executed
} while (condition);
Example:
int num = 1;
do {
System.out.println("Value of num: " + num);
num++;
} while (num <= 5);
Nested Loops
Java allows you to nest loops, which means placing one loop inside another. This is useful for more complex scenarios where you need to iterate through multiple dimensions of data.
Example of nested loops:
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 2; j++) {
System.out.println("i: " + i + ", j: " + j);
}
}
Loop Control Statements
break Statement
The break
statement allows you to exit a loop prematurely, typically when a certain condition is met.
continue Statement
The continue
statement skips the current iteration of a loop and proceeds to the next iteration.
Read more about Conditional Statement
Advantages of Using Loops
Code Reusability
Loops promote code reusability by allowing you to perform the same action on multiple items or execute the same block of code multiple times.
Efficient Data Processing
Looping is essential for processing large datasets, making calculations, or searching for specific elements within an array or collection.
Enhanced Readability
Using loops can lead to more readable code, especially when dealing with repetitive tasks, as it reduces redundancy.
Real-Time Practical Example: Calculating Fibonacci Series
Real-Time Practical Example: Calculating Fibonacci Series
In this section, we’ll walk through a practical example of using loops in Java to calculate the Fibonacci series. The Fibonacci series is a sequence of numbers where each number is the sum of the two preceding ones, typically starting with 0 and 1.
Problem Statement
Let’s write a Java program to generate the Fibonacci series up to a specified number of terms. We’ll use a loop to calculate each term in the series and print the result.
Code Walkthrough
public class FibonacciSeries {
public static void main(String[] args) {
int n = 10; // Number of terms in the Fibonacci series
long firstTerm = 0;
long secondTerm = 1;
System.out.println("Fibonacci Series up to " + n + " terms:");
for (int i = 1; i <= n; i++) {
if (i == 1) {
System.out.print(firstTerm + " ");
continue;
}
if (i == 2) {
System.out.print(secondTerm + " ");
continue;
}
long nextTerm = firstTerm + secondTerm;
System.out.print(nextTerm + " ");
firstTerm = secondTerm;
secondTerm = nextTerm;
}
}
}
Output:
Fibonacci Series up to 10 terms:
0 1 1 2 3 5 8 13 21 34
In this Java program, we first specify the number of terms we want in the Fibonacci series (in this case, 10 terms). We then initialize the first and second terms of the series as 0 and 1, respectively.
Inside the for
loop, we calculate each subsequent term by adding the previous two terms. We use a continue
statement to handle the first two terms, as they are predefined.
As the loop iterates, it prints each term of the Fibonacci series up to the specified number of terms, resulting in the sequence 0 1 1 2 3 5 8 13 21 34
.
This example demonstrates how loops in Java can be used to perform repetitive calculations and generate sequences, making them a powerful tool for solving a wide range of programming problems.