3. Mastering Spring: Unleash the Power of Stereotype Annotations in Spring Framework
Stereotype Annotation Hierarchy:
The stereotype annotations are generally used to create bean(instance) of the Class.
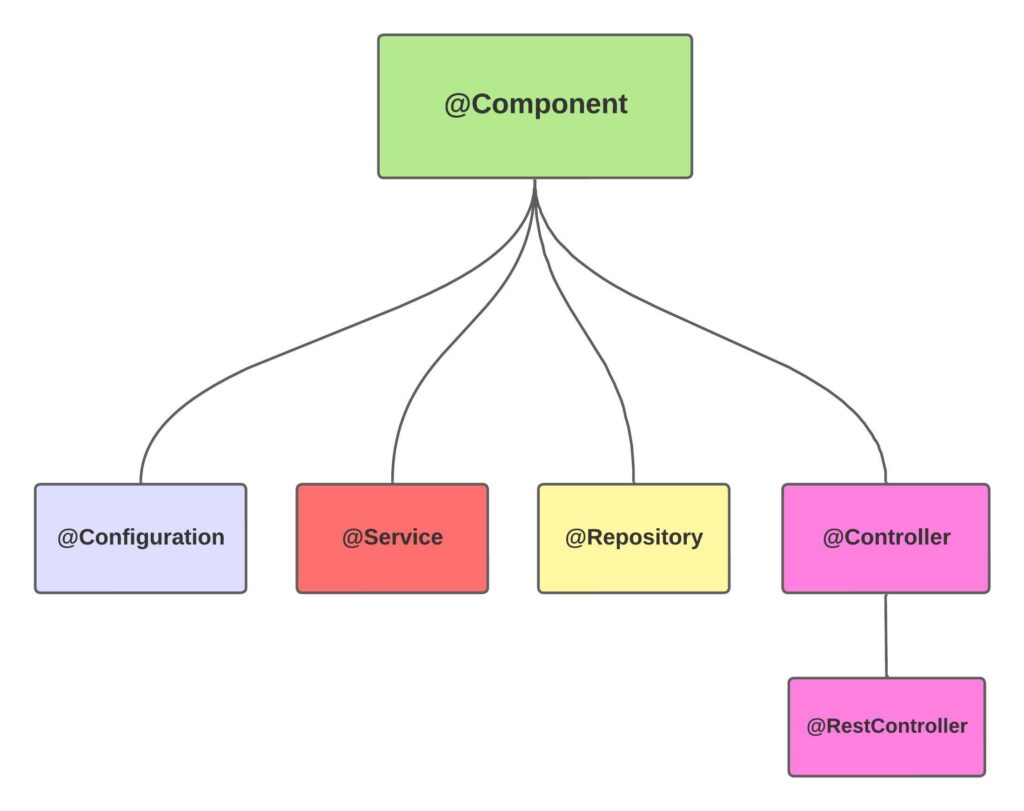
@Component: – It is a class level annotation. It is used to mark a Java class as a bean. A Java class annotated with @Component is found during the class path. The spring framework pick it up and configure it in the application context as a Spring Bean.
@Configuration: – It is a class level annotation. The class annoted with @Configuration used by spring Containers a source of bean definitions.
@Repository: –It is a class level annotation. @Repository annotation is a specialization of @Component annotation which is used to indicate that the class provides the mechanism for storage, retrieval, update, delete and search operation on objects. The repository is a DAOs (Data Access Object) that access the database directly. Though it is a specialization of @Component annotation, so Spring Repository classes are autodetected by spring framework through classpath scanning.
@Service: – It is class level annotation. It is also a specialization of @Component Annotation like the @Repository Annotation. It tells the spring that class contains the business logic.
@Controller: – It is class level annotation. it is also specialization of @Component Annotation. It marks a class as a web request handler. It is often used to serve web pages. By default, it returns as String that indicates which route to redirect. It is mostly used with @RequestMapping annotation.
@RestController: – It can be considered as a combination of @Controller and @ResponseBody annotations. The @RestController annotation is itself annoted with @ResponseBody annotation. It eliminates the need for annoting each method with @ResponseBody.
Spring Boot Annotations
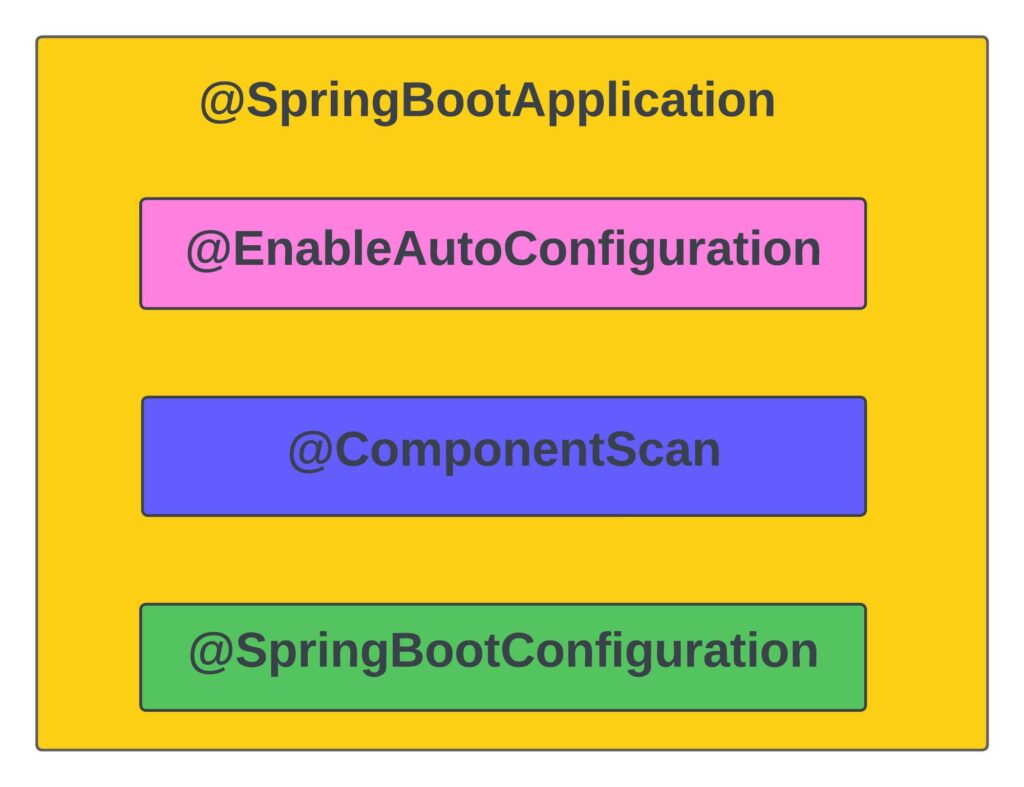
@SpringBootApplication :- @SpringBootApplication annotation is combination of three annotations @EnableAutoConfiguration, @ComponentScan, and @SpringBootConfiguration.
@ComponentScan: – The component scan annotation is scan all the classes in package and its sub packages and create beans if the class is annotated with stereotype annotations.
Spring MVC And REST Annotations
@RequestMapping :- It Is used to map the web requests . It has many optional elements like consumes , header , method , name , params , path , produces , and values . We use it with the class as well as the method.
@GetMapping :- It maps the HTTP GET requests on the specific handle method . It is used for data RETRIEVE.
@PostMapping: – It maps the HTTP POST request on the specific handler method. It is used for ADD.
@PutMapping: –It maps the HTTP PUT request on the specific handler method. It is used for UPDATE.
@DeleteMapping: – It maps the HTTP DELETE requests on the specific handler method. It is used for DELETE.
@RequestBody: – It is used to bind HTTP request with an object in a method parameter. Internally it uses HTTP Message Converter to convert the body of the request. When we annotate a method parameter with @RequestBody, the spring framework binds the incoming HTTP request to the body parameters.
@ResponseBody: –It binds the method return value to the response body. It tells the spring boot framework to serialize a return an object into JSON and XML format.
@PathVariable: – It is used to extract the values from the URL. It is most suitable for the RESTful web service, where the URL contains a path variable. We can define multiple @PathVariable in a method.
@RequestParam: – It is used to extract the Query parameters from the URL. It is also Known as Query parameter. It can specify default values if the query parameter is not present in the URL.
@RestController: – It can be considered as a combination of @Controller and @ResponseBody annotations. The @RestController annotation is itself annoted with the @ResponseBody annotation. It eliminates the need for annoting each method with @ResponseBody.
@RequestMapping And Its Attributes
The @RequestMapping annotation is a core annotation in Spring Boot that is used to map a specific HTTP request method to a specific Java method in web application. This means that when a request is sent to the web application, Spring Boot will use the @RequestMapping annotation to determine which Java method should handle the request.
The @RequestMapping annotation can be used to specify the request type like GET, POST, PUT, DELETE this will handle the request. for example, if you want to handle GET request to the “/contact” URL path, there is two ways to handle specific request you can use @RequestMapping (value = “/contact”, method=RequestMethod.GET) this will tell Spring Boot that this Java method should handle GET request to the “/contact” path. You can also use @GetMapping (value = “/contact”) both annotation handle GET request. In @GetMapping annotation there is @RequestMapping annotation which is working Internally like this @RequestMapping (method = RequestMethod.GET).
The @RequestMapping annotation is commonly used in RESTful web services to map HTTP request methods to specific Java methods that handle those requests. For example, a GET request to the /contact URL path can be used to retrieve the list of contact from a database, while POST request to the same URL path can be used to create new contact in the database. Additionally, Spring Boot provides other related annotations, such as @GetMapping, @PostMapping, @PutMapping, @DeleteMapping. This annotation has specific HTTP methods in @RequestMapping.
Attributes of @RequestMapping Annotation
When using the @RequestMapping annotation at method-level in Spring Boot application, the annotation provides a number of attributes that can be used to customize the behavior of request mapping. Some of the commonly used attributes are:
->value: This attribute specifies the URL path that the request mapping should apply to. for example, @RequestMapping(“/contact”) would match any request with the URL path “/contact”.
->method: This method specifies the HTTP method that the request mapping should apply to. It can take a RequestMethod value such as GET, POST, PUT, DELETE, etc. For example, @RequestMapping (values=”/contact”, method=RequestMethod.GET) would match any GET request with URL path “/contact”.
->params: This attribute specifies the request parameter conditions that should be met for the request mapping to be considered a match. For example, @RequestMapping (value = “/contact”, params = “id“) would match any request with URL path of “/contact” that has an “id” parameter.